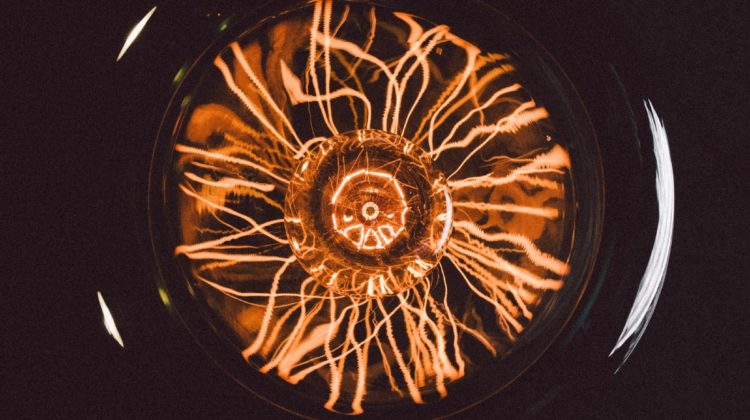
We can solve this prompt using a for loop as follows:
def add(num):
# if num is an integer then
if type(num) == int:# create sum variable and assign it to 0
sum = 0# using a for loop, loop over integers 0 to num
for x in range(num+1):# update sum value
sum += x# return sum
return sum# if num is not an integer then return 0
else:
return 0
Let’s dissect the code below.
We first check if the value passed in, num, is an integer using the type function.
if type(num) == int:
If the type is an integer, we create a sum variable and assign it the value 0.
sum = 0
We then loop over the integers starting from 0 through the integer passed in to our function using a for loop and the range function. Remember that the range function creates a range object, which is an iterable object, that starts at 0 (if we don’t specify a start value), and goes to the integer less than the stop value (since the stop value is exclusive). That is why we need to add 1 to the stop value (num+1), since we want to add up all the integers from 0 up to and including that number, num.
range
(start, stop[, step])
The range function will create a range object, which is an iterable object, and thus we can use a for loop to loop through it. As we are looping through this iterable object, we are adding each number, or x, to the sum variable.
for x in range(num+1):
sum += x
Then after the for loop iterations are complete, the function returns the sum.
return sum
Lastly, if the number passed in is not an integer, we return 0.
else:
return 0