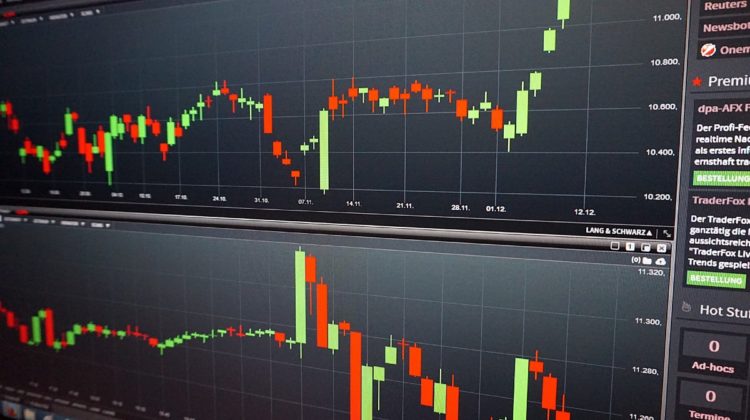
We have 8 indicators that we want to turn into just one technical indicator. For simplicity, we will give each indicator an equal weight of 12.5%. The way we will do this is normalizing the indicators that need normalization, multiplying them later by 12.5% (their weight), and finally, adding the pieces together. Here is a quick summary of what we will do:
- The Relative Strength Index is already normalized and therefore no transformation is needed. We can simply multiply its values by 12.5%. As an example, if the RSI is showing a value of 100, then multiplying it by 12.5% will give a reading of 12.5 which is the maximum value of a weighted component in the Global Technical Index. This pushes the GTI up to approach a bearish zone.
my_data[:, rsi_column] = my_data[:, rsi_column] * 0.125
- The Stochastic Oscillator is already normalized and therefore no transformation is needed. We can simply multiply its values by 12.5%. As an example, if the Stochastic is showing a value of 0, then multiplying it by 12.5% will give a reading of 0 which is the minimum value of a weighted component in the Global Technical Index. This pulls the GTI down to approach a bullish zone.
my_data[:, stoch_column] = my_data[:, stoch_column] * 0.125
- The Rate of Change Indicator needs to be normalized with values between 0 and 100 and then, we can simply multiply its values by 12.5%. The normalization function is shown below.
- The Momentum Indicator needs to be normalized with values between 0 and 100 and then, we can simply multiply its values by 12.5%. The normalization function is shown below.
- The Modified Fisher Transformation needs to be normalized with values between 0 and 100 and then, we can simply multiply its values by 12.5%. The normalization function is shown below.
- The MACI is already normalized and therefore no transformation is needed. We can simply multiply its values by 12.5%.
- The Normalized Index is actually the function itself and can be seen as a High-Low index with a selected lookback period, therefore, we will simply multiply by 12.5% to get its weight.
- The Astral Pattern: As it is composed of values from -8 to +8 with -8 as a bullish timing signal and +8 as a bearish timing signal, we can simply create gradual values from 0 to 100 with 6.25 for every step. For example, -8 becomes 0, -3 becomes 31.25, 0 becomes 50, +7 becomes 93.75, and +8 becomes 100.
Let us quickly discuss how we normalize values between 0 and 100 before we proceed to understand more the GTI.
This great technique allows us to trap the values between 0 and 1 (or 0 and 100 if we wish to multiply by 100). The concept revolves around subtracting the minimum value in a certain lookback period from the current value and dividing by the maximum value in the same lookback period minus the minimum value (the same in the nominator).
We can try to code this formula in python. The below function normalizes a given time series of the OHLC type:
def normalizer(Data, lookback, what, where):for i in range(len(Data)):
try:
Data[i, where] = (Data[i, what] - min(Data[i - lookback + 1:i + 1, what])) / (max(Data[i - lookback + 1:i + 1, what]) - min(Data[i - lookback + 1:i + 1, what]))except ValueError:
passData[:, where] = Data[:, where] * 100
return Data# The what variable is what to normalize and the where variable is where to print the normalized values, i.e. which colum.