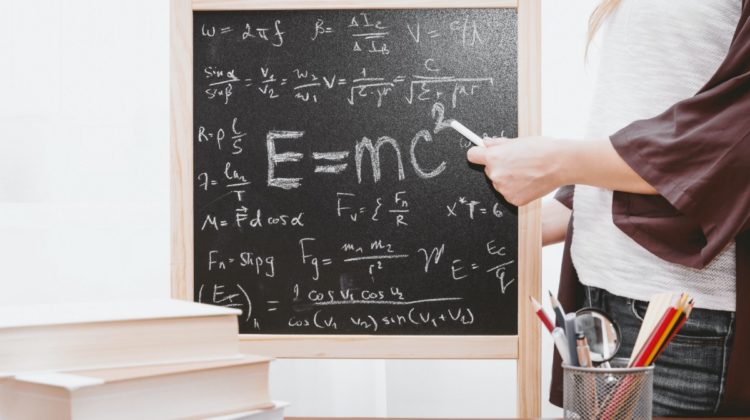
– A strobogrammatic number is a number that looks the same when rotated 180 degrees (looked at upside down).
– Write a function to determine if a number is strobogrammatic. The number is represented as a string.
– https://leetcode.com/problems/strobogrammatic-number/
Walk Through My Thinking
Facebook asks this question. It would be helpful to write out some numbers and rotate them 180 degrees.
Original 180 degrees rotation
0 0
1 1
2 invalid
3 invalid
4 invalid
5 invalid
6 9
7 invalid
8 8
9 6
From 0 to 9, there are only 5 valid digits and 5 invalid digits after 180-degree rotation. For these valid digits, only 0, 1, and 8 stay the same after rotation, and the other two, 6 and 9, change values.
On the one hand, it would not be a valid number after rotations if the number contains any invalid digits.
On the other hand, we return the “mirrored” value for the valid digits (0, 1, 6, 8, and 9). We can create a dictionary and take advantage of its key-value pair feature to return the value.
Dictionary has a unique key-value pair feature, which comes in handy under a lot of scenarios. Take a look here:
Solution
In Python, we can do the following:
True
There are two catches. First, num is stored as a string, not an integer, and we have to be careful with accessing the string element. Second, digits change their positions after rotations, and so we have to use the reversed() method to read from the last (rightmost) digit backward, e.g., 169 → 691.
The rest is self-evident.