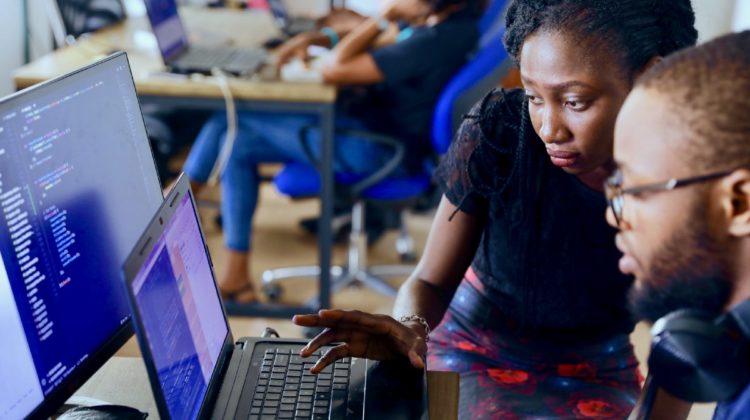
Learn how to do local machine like development and debugging on the cloud (remote instances) using Visual Studio Code for Deep Learning.
Before joining the industry, when I was working as a Graduate Researcher in the Vision and Image Processing Lab at the University of Waterloo, I had access to the beast NVIDIA® GeForce® RTX 2080 Ti locally. So I was able to train models locally without needing to use compute instances on the cloud. (Sadly I didn’t work on models that required multi GPU training.) However, after joining Fugro as a Deep Learning/ Computer Vision Engineer, I was exposed to AWS EC2 instances and S3.
Development on the cloud is a completely different experience compared to just using a laptop/desktop. I’ve learnt a lot about it from my experience and want to share it so that you may benefit from it too. Remote development is an essential skill to have and is all the more invaluable in today’s world when most of us (who work in remote work compatible jobs) are working from home. I hope after reading this article you feel motivated to try using this remote development approach in your workflow to make your life a bit easier.
Tip: I’ve thoroughly tested and evaluated both AWS SageMaker Studio (based on Jupyter Lab) and the Databricks platform (surprisingly based on the older Jupyter Notebook). I found the remote development using Visual Studio to be the most productive, flexible, and time-saving. For Computer Vision/Deep Learning researchers/engineers, I absolutely recommend using VS Code Remote Development.
Let us start by looking at a few differences between remote and local development.
I used to use Remote Desktop Software for development. (I personally preferred AnyDesk over TeamViewer.) But setting these up on Linux compute instances may be challenging. More importantly, since they stream video, you need a mighty powerful broadband connection at both ends. This approach can become sluggish really quickly. Finally, although this kind of software is usually free for individual/personal use, you need to purchase a license for commercial usage.
For this article, I am using the lifetime free compute instance from Oracle. (Note: You get 100 GB free lifetime storage which can be easily used as a backup drive for your documents or other files. This is what motivated me to create an account in the first place.) One of my colleagues had shared this with me and one good gesture deserves another, so here I am, sharing this with you.
For these cloud instances typically SSH is installed, configured and enabled by default. I won’t be covering server setup and configuration, SSH key generation and your public key addition to the server, since that is well beyond the scope of this article. However, if you find yourself wanting to set it up for your own machine, you can refer the following guides.
No matter which cloud platform you decide to use, you’ll require the following information to remotely connect to your machine.
- Public IP Address: This is the IP address which we will use to connect to the instance. (Note: You can use a private IP with a subnet group, however, you’ll have to ensure that you are connected to the necessary VPN to be able to access your machine.) e.g. 123.123.123.123
- Username: The username for your instance would be required for login.
- SSH private key: This is the SSH key that is normally generated during the instance creation. It would be something like oracle-ssh-key, aws-ssh-key.pem etc.
Let us now look at remote development on VS Code.
For those of you that do not know about the VS Code, it is a cross-platform open-source code editor by Microsoft. I have tried Eclipse, PyCharm, Sublime, Spyder, Notepad++, Atom and Microsoft Visual Studio, but none of them came close to VS Code. ( This is my personal choice, you may have a different opinion and I respect that. )
Please download and install the following software on your local machine.
- VS Code: https://code.visualstudio.com/
- Miniconda: https://docs.conda.io/en/latest/miniconda.html ( This is a much smaller installation compared to Anaconda. As a result this leads to lesser bloat on your system. )
After opening VS Code please install the following extensions. ( [Required] — Means required for this article, [Recommended] — Extension that I recommend for your setup. )
- Visual Studio Code Remote Development Extension Pack: This will install the extensions required for remote development. [Required] This includes the following three extensions.
- Remote-SSH – Work with source code in any location by opening folders on a remote machine/VM using SSH. Supports x86_64, ARMv7l (AArch32), and ARMv8l (AArch64) glibc-based Linux, Windows 10/Server (1803+), and macOS 10.14+ (Mojave) SSH hosts.
- Remote-Containers – Work with a sandboxed toolchain or container-based application by opening any folder mounted into or inside a container.
- Remote-WSL – Get a Linux-powered development experience from the comfort of Windows by opening any folder in the Windows Subsystem for Linux.
Note: You can choose to install only Remote — SSH if you know you won’t be working with containers or WSL.
After installation, your VS Code will have a new icon in the left panel called the Activity Bar.
2. Python extension for Visual Studio Code: A must-have Python extension for Visual Studio. [Required]
3. Pylance: Great for python static type checking. [Recommended]
4. Jupyter: Extension for basic notebook support. [Recommended]
5. Visual Studio IntelliCode: Good for code suggestions. [Recommended]
6. Git Graph: This extension allows you to view Git Graph of your repository, and to easily perform Git actions from the graph. [Recommended]
7. Python Docstring Generator: For quickly generating properly formatted docstrings for python functions. [Recommended]
8. Python Test Explorer for Visual Studio Code: For running Python Unittest or Pytest tests with the Test Explorer UI. [Recommended]
9. GitHub VS Code theme: Simple dark and light GitHub themes. [Recommended]
10. Markdown Preview Enhanced: Great utility if you work with markdowns or for readme files. [Recommended]
11. VS Code Icons: Brings icons for your file explorer. [Recommended]
Now that we have our local software setup, let us briefly look at how the VS Code Remote Development works.
VS Code Remote Development allows you to use a container, remote machine, or the Windows Subsystem for Linux (WSL) as a full-featured development environment. Few highlights include:
- Develop on the same operating system you deploy to or use larger or more specialized hardware.
- Sandbox your development environment to avoid impacting your local machine configuration.
- Make it easy for new contributors to get started and keep everyone on a consistent environment.
- Use tools or runtimes not available on your local OS or manage multiple versions of them.
- Develop your Linux-deployed applications using the Windows Subsystem for Linux.
- Access an existing development environment from multiple machines or locations.
- Debug an application running somewhere else such as a customer site or in the cloud.
No source code needs to be on your local machine to get these benefits. Each extension in the Remote Development extension pack can run commands and other extensions directly inside a container, in WSL, or on a remote machine so that everything feels like it does when you run locally.
VS Code sets up a server on your remote machine during the first initialization/setup. This server is then used for executing all your commands and extension functionalities directly on the remote instance. All it needs is an SSH connection to the server. So you don’t need to keep multiple copies of the code. Instead, you can do direct development on the remote machine with all the VS Code functionalities.
Now, we look at how to connect to the remote host using VS Code.
You will need to use the information that I asked you to get in the Introduction. We construct an SSH command as follows. [Note: You may need to create the .ssh folder in your system. The Username and the Public IP Address can be found from the instances section in your console. The key would be generated during the instance creation.
ssh -i “C:Usersjanedoe.sshoracle-ssh-key” username@your-public-ip-address
The -i flag selects a file from which the identity (private key) for public key authentication is read. Note: Put the key path in double-quotes for Windows.
Now we’ll use this command to create a connection to our remote instance. In the video, I show how to edit and run a python script on your remote instance. However, the connection steps are also shown below.
Once you have installed the remote extension, a new indicator would be visible at the bottom left corner of the status bar. This indicator tells you in which context the VS Code is running (local or remote).
- Click on the indicator to bring up a list of Remote extension commands.
2. Choose the Remote-SSH: Connect to Host… command and connect to the host by entering ssh -i “C:Usersjanedoe.sshoracle-ssh-key” username@your-public-ip-address.
VS Code will now open a new window (instance). You’ll then see a notification that the “VS Code Server” is initializing on the SSH Host. Once the VS Code Server is installed on the remote host, it can run extensions and talk to your local instance of VS Code.
You will know you’re connected to your remote instance by looking at the indicator in the Status bar. It shows the hostname of your Virtual Machine.
You are now connected to your host at this point!
The Remote — SSH extension also contributes a new icon on your Activity bar, and clicking on it will open the Remote Explorer. From the dropdown, select SSH Targets, where you can configure your SSH connections. For instance, you can save the hosts you connect to the most and access them from here instead of entering the user and hostname.
Once you’re connected to your SSH host, you can interact with files and open folders on the remote machine. If you open the integrated terminal (Ctrl+`), you’ll see you’re working inside a bash shell while you’re on Windows.
You can use the bash shell to browse the file system on the VM. You can also browse and open folders on the remote home directory with File > Open Folder.
A more in-depth tutorial can be found here: https://code.visualstudio.com/docs/remote/ssh-tutorial
Finally, let us look at how to use the VS Code debugger on the remote machine.
Debugging in a remote session is exactly the same as a local connection. You can inspect variables, add watch variables, add breakpoints, have access to the call stack if an Exception is thrown. In the following video, I demonstrate the key debugging functionalities that you may commonly use in your development process.
For a more in-depth dive please read the official documentation here: https://code.visualstudio.com/docs/python/debugging
In this post, I covered how to use VS Code for Remote Development on any Virtual Machine or Remote Instance via SSH. We also looked at how to do remote debugging. I have tried and tested SageMaker Studio and Databricks, but none of them will offer such flexibility. You can set up your own custom images, environments and work as if you are on a local machine. If you are working on Deep Learning and working on research then this would prove to be an invaluable capability. And the beautiful thing about this is that VS Code is free (open source). I hope this helps you in your work and that you enjoyed reading my article as much as I loved writing it! Thank you for reading the article!
[1] https://code.visualstudio.com/docs/remote/remote-overview