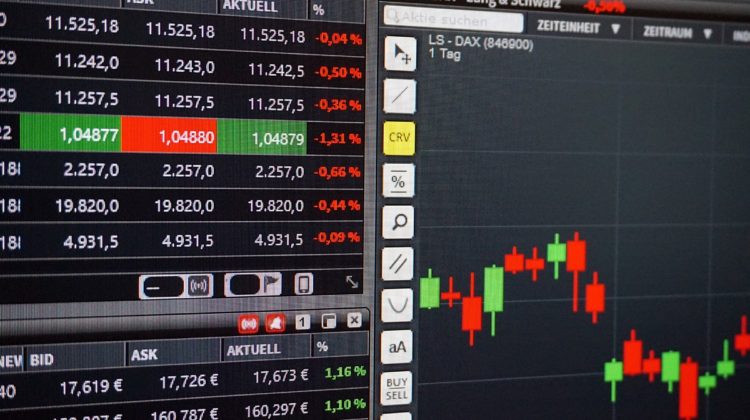
Now, we can finally and easily code this in Python this way:
def demarker(Data, lookback, high, low, where): # Calculating DeMAX
for i in range(len(Data)):
if Data[i, high] > Data[i - 1, high]:
Data[i, where] = Data[i, high] - Data[i - 1, high]
else:
Data[i, where] = 0
# Calculating the Moving Average on DeMAX
Data = ma(Data, lookback, where, where + 1)
# Calculating DeMIN
for i in range(len(Data)):
if Data[i - 1, low] > Data[i, low]:
Data[i, where + 2] = Data[i - 1, low] - Data[i, low]
else:
Data[i, where + 2] = 0
# Calculating the Moving Average on DeMIN
Data = ma(Data, lookback, where + 2, where + 3)
# Calculating DeMarker
for i in range(len(Data)):
Data[i, where + 4] = Data[i, where + 1] / (Data[i, where + 1] + Data[i, where + 3])
# Removing Excess Columns
Data = deleter(Data, where, 4)
return Data
For the above function to work, we need an OHLC array containing a few spare columns, the moving average function, and the deleter function. Here is how to do that:
def adder(Data, times):for i in range(1, times + 1):
z = np.zeros((len(Data), 1), dtype = float)
return Datadef deleter(Data, index, times):
Data = np.append(Data, z, axis = 1)for i in range(1, times + 1):
Data = np.delete(Data, index, axis = 1)
return Datadef ma(Data, lookback, what, where):for i in range(len(Data)):
try:
Data[i, where] = (Data[i - lookback + 1:i + 1, what].mean())except IndexError:
pass
return Data