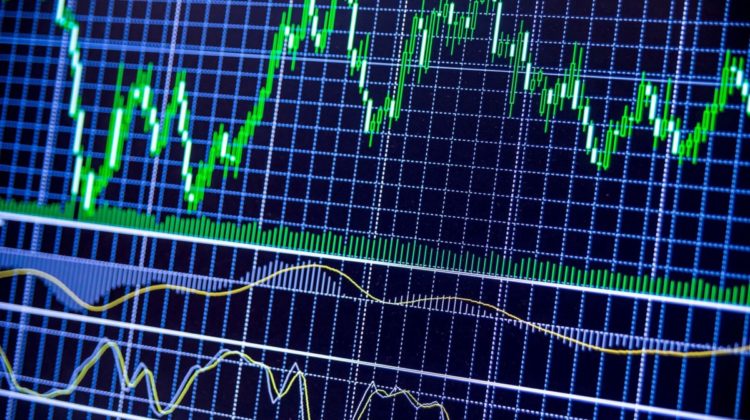
Before proceeding with the indicator’s code, we have to code two simple functions:
def deleter(Data, index, times):for i in range(1, times + 1):
Data = np.delete(Data, index, axis = 1)return Data
def jump(Data, jump):
Data = Data[jump:, ]
return Data
Now, we are ready for the code. Remember to have an OHLC array ready. You can see how to import an OHLC automatically in a previous article I have posted.
def fibonacci_rsi(Data, high, low, close, where):# Taking price differences
Data = adder(Data, 6)for i in range(len(Data)):
Data[i, where] = Data[i, high] - Data[i - 1, high]
for i in range(len(Data)):
Data[i, where + 1] = Data[i, low] - Data[i - 1, low]
Data[:, where + 2] = 0.5 * (Data[:, where] + Data[:, where + 1])
Data = deleter(Data, where, 2)
# Finding up and down columns
for i in range(len(Data)):if Data[i, where] > 0:
if Data[i, where] < 0:
Data[i, where + 1] = Data[i, where]
elif Data[i, where] < 0:
Data[i, where + 1] = 0
Data[i, where + 2] = Data[i, where]
elif Data[i, where] > 0:
Data[i, where + 2] = 0Data[:, where + 2] = abs(Data[:, where + 2])
# Taking the Fibonacci Moving Average
for i in range(3, 9):Data = adder(Data, 1)
lookback = fib(i)
Data = ma(Data, lookback, where + 1, -1)Data = adder(Data, 1)
Data = jump(Data, lookback)for i in range(len(Data)):
Data[i, -1] = np.sum(Data[i, where + 3:where + 9 - 3])
Data[i, -1] = Data[i, - 1] / (9 - 3)Data = deleter(Data, where + 3, 9 - 3)
for i in range(3, 9):Data = adder(Data, 1)
lookback = fib(i)
Data = ma(Data, lookback, where + 2, -1)Data = adder(Data, 1)
Data = jump(Data, lookback)for i in range(len(Data)):
Data[i, -1] = np.sum(Data[i, where + 4:where + 9 - 3])
Data[i, -1] = Data[i, - 1] / (9 - 3)Data = deleter(Data, where + 4, 9 - 3)
# Calculating the Fibonacci RSI
Data = adder(Data, 2)Data[:, where + 5] = Data[:, where + 3] / Data[:, where + 4]
for i in range(len(Data)):
Data[i, where + 6] = (100.0 - (100.0 / (1.0 + Data[i, where + 5])))
Data = deleter(Data, where, 6)
return Data
We can place the oversold/overbought barrier at 15/85 from where we can start thinking about fading the move.