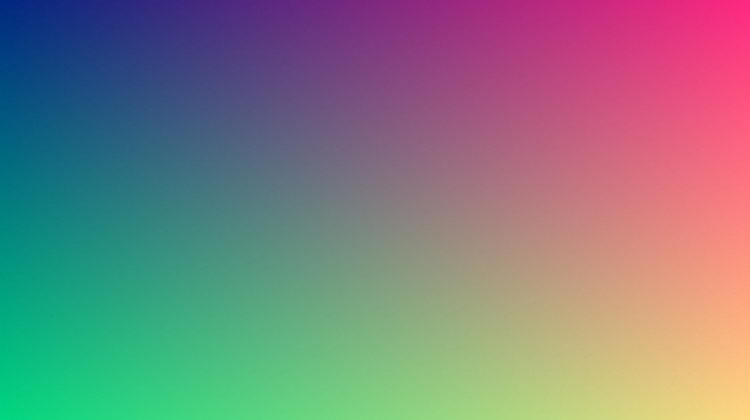
To code the Rainbow Indicator, we can use the following code on an OHLC array with a few columns to spare.
def rainbow(Data, ma1, ma2, ma3, ma4, ma5, ma6, ma7, what, where): # Converting Exponential lookback to Smoothed Lookback
ma1 = (ma1 * 2) - 1
ma2 = (ma2 * 2) - 1
ma3 = (ma3 * 2) - 1
ma4 = (ma4 * 2) - 1
ma5 = (ma5 * 2) - 1
ma6 = (ma6 * 2) - 1
ma7 = (ma7 * 2) - 1
# Calculating the Smoothed Moving Averages A.K.A The Rainbow Moving Average
Data = ema(Data, 2, ma1, what, where)
Data = ema(Data, 2, ma2, what, where + 1)
Data = ema(Data, 2, ma3, what, where + 2)
Data = ema(Data, 2, ma4, what, where + 3)
Data = ema(Data, 2, ma5, what, where + 4)
Data = ema(Data, 2, ma6, what, where + 5)
Data = ema(Data, 2, ma7, what, where + 6)
# The Rainbow Oscillator
Data[:, where + 7] = Data[:, where] - Data[:, where + 6]
return Data
To add a specified number of empty columns into an array, we can use the below adder function:
def adder(Data, times):for i in range(1, times + 1):
z = np.zeros((len(Data), 1), dtype = float)
return Data
Data = np.append(Data, z, axis = 1)