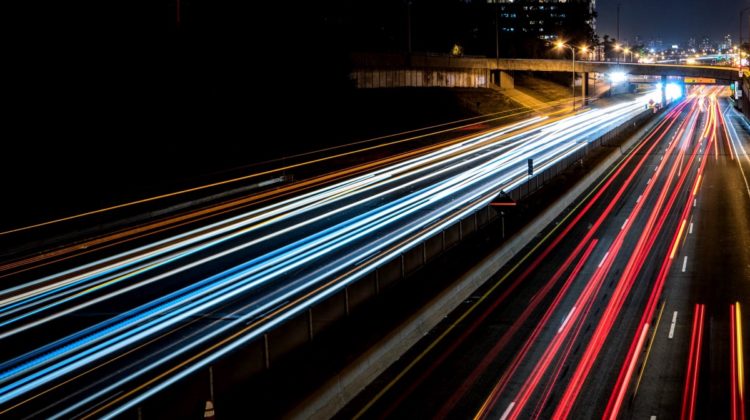
In the past years, Python has proven itself an easy to learn, versatile, and potent programming language. It became one of the top choices to learn if you’re new to the programming world or if you’re interested in scientific programming, data science, or general computer science.
Due to this increase in popularity, developers and companies utilized Python in their daily work routine by using it to build applications, conduct research, and develop packages for others to use. Now, there are more than 200,000 Python packages out there covering a wide range of applications from simple string manipulation to full-on machine learning and artificial intelligence packages.
Python is the complete framework for anyone wanting to get into programming in general or any data science branch in particular. Python offers many advantages to help people build their portfolio and a career. After all, once you mastered a programming language, learning another is not that complex of a task.
Although Python’s advantages outweigh its disadvantages, one disadvantage seems to be the center of many discussions when someone argues against Python, “Python is too slow.” Speed is essential to all of us these days; technology had made us impatient; if an app took a second longer to open or a webpage took a couple of seconds to load, we got frustrated because it’s too slow.
The truth is, Python is relatively slow compared to other programming languages. There’re various reasons for Python slowness, but the main one is its nature as an interpreted language. And that’s something that we can’t change. So how can we speed up Python?
There are simple steps we can make while writing our code that results in faster execution. Maybe not as fast as C, C++, or Java, but fast enough for Python code to make it better.
Python developers are working hard to keep Python relevant, potent and implement its core functionalities in a more efficient way for future releases. Keeping your code up-to-date by using the latest Python release will ensure that you’re using new optimization techniques implemented in the release.
So, planning your next step using the latest release is always a good idea as long as this release is compatible with all your dependencies.
Staying up-to-date also applies to the packages and libraries you’re using. The library providers are always working on fixing bugs, adding new features, and optimizing the existing ones.
Data structures are the fundamental building blocks of computer science. There are many different data structures implemented in Python, such as lists, tuples, dictionaries, sets, and more. But, often, people tend to keep using lists for all their iterable tasks.
Knowing the correct data structure to use for your application can make it much faster. For example, using dictionaries and sets is more efficient in search applications than lists because they are built based on hash tables and always require O(1)
time to look up any item.
Making sure that you’re using the proper data structure is a skill that requires you to know more about the different types of data structures and the best application for each one of them.
Loops are an essential part of any programming language; no code’s free of loops. But, loops often take up the most time in execution. Sometimes, we overdue it in using loops, which ends up making our code significantly slower.
Hence, we need to decrease the amount of looping in our code. That can be done using a different variable to sort a list instead of looping through the list or using refactoring techniques like intersection and unions to optimize our code.
Another way to use loops more efficiently is to use list comprehension whenever possible; for example, creating and filling up a list is better done through comprehension than using the append method. Finally, while
loops are more static and time-efficient than for
loops, when possible, replace for loops with while loops.
Python offers so many built-in functions, and those that are not offered are often provided using some package developed by Python developers or any other company. So, use what’s offered instead of rebuilding it.
I see many people who like to implement functions themselves, nothing wrong with that if you’re learning the language or just doing so to expand your programming knowledge. But, if you’re using Python to build a large application, implementing most functionality that is already there may be a waste of time and cause your code to be significantly larger and slower.
Built-in functions like sum
, max
, any
, filter
, and map
are implemented using C, making them fast and efficient. Moreover, the Python collection module offers a wide variety of data structures that you can use right away to optimize your code. Always look up what’s there before you start building it.
One of the things that take up a lot of execution time is memory interactions. So, using some memory-saving techniques can make your code noticeably faster. There are different approaches you can use to save up memory time, including:
- Use generators to generate range values because they require less memory usage.
- Use
sort()
instead ofsorted()
because sorted creates a new copy of the iterable. Using sorted is more efficient in the case of large datasets. - Use slots. The
__slots__
is a special way of declaring variables that take the instance of a variable and reserves only enough space for its value to be held. - Use math and Numpy to deal and perform operations on large numbers and matrices. These libraries are built to be memory efficient.
- Use the
format
orjoin
methods to concatenate strings instead of using the+
operator.
Various applications can be used to speed up your Python code. These applications, however, require you to rewrite parts of your code to make it more efficient.
For example, you can use CPython
to write parts of your code using C or use C functions. The code generated by CPython is compiled into machine language, making it faster to execute. Another choice is to use Numba
, which is a just-in-time compiler. Numba basically turns Python code into a faster machine code for faster execution.
PyPy
is also an option to speed up your code. It provides a good alternative to CPython. The only downside to PyPy is that it’s not compatible with all Python packages, so ensure all your dependencies are supported before you decide to use PyPy.
Python is one of the most popular programming languages out there; it’s easier to learn for beginners and professionals, simple to read and comprehend, and can be used in almost every application you can think of. In 2019, SlashData estimated the number of developers using Python as their main programming language to 8.2 million developers, with numbers going up every day.
Python’s many advantages are what kept it alive all these years and what encouraged many developers and companies to build and develop many packages that anyone can use to build and deploy applications from a wide range of fields.
Despite its success, there’s always one disadvantage that is always held against Python, its slowness. Since Python is an interpreter-based language, it defaults slower than compiled languages because it executes and runs the code line by line.
We can’t change Python’s nature, but we can implement simple steps when writing our code relatively faster and more efficiently. In this article, we went through 6 ways you can use to make your Python code faster. That’s not to say that these are the only ways to speed up your code, but they’re a great way to start optimizing your codebase.