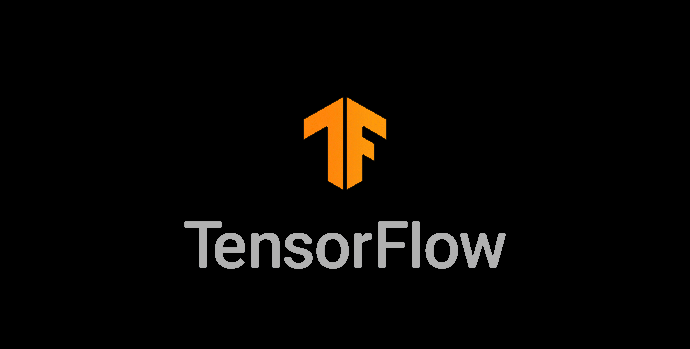

In this article I will be covering the basics of TensorFlow that you need to understand before going deep into TensorFlow. The topics include what is a tensor, constant and variable.
TensorFlow is an open-source library for machine learning developed by Google. TensorFlow consist of both High-level API and Low level API .
High-level API provides more functionality within one command as compared to lower-level API but Low-Level API allows user to have more detailed control to manipulate funtions.
Eager execution is enabled by default therefore it allows user to write simpler and more intuitive code. Moreover TensorFlow model building is now centered around the Keras and Estimators high-level APIs
According to the TensorFlow documentation a tensor is “a generalization of vectors and matrices to potentially higher dimensions.”. If you do not understand you can simply think of it as a collection of numbers which is arranged into a particular shape.
We will learn how to define tensor by defining 1, 2 and 3 dimensional tensors.
Before we define a tensor we need to import the necessary package.
import tensorflow as tf
1 dimensional tensor
tf.ones(2,)
2 dimensional tensor
tf.ones((2,2))
3 dimensional tensor
tf.ones((2,3,4))
The parameter needed to create ones is shape , in this case we are going to be using the 3 dimensional tensor example. We can think of the 2 in the bracket as row then the 3 as columns and 4 as thickness like a cube . Referring back to the 1d tensor it will simply be a tensor with only rows.
Do note that, you can also create more then 3 dimensional tensor.
Constant is the simplest category of tensor in tensor. A constant is something that cannot be trained to put it into simpler terms a constant is something that cannot be changed after defining it. However it can have any dimension.
How to define a constant ?
#this is a 2x3 tensor made of 2s
constant(2,shape[2,3])
There are certain situations when they are more convenient options for defining certain type of special tensors , previously we used tf.ones to generate an arbitrary dimension that is populated with ones. Additionally we can also generate a tensor populated with zeros as shown below.
tf.zeros(2,2)
Another function that will be useful is zeros_like or ones_like , we can use this function on a tensor to populate it with zeros or ones copying the dimensions of the input tensor. An example is provided below
#first we define a constant
c = tf.constant([[1, 2, 3], [4, 5, 6]])
tf.zeros_like(c)
Lastly is the fill function which can be used to fill a tensor with desired number of dimension with the same value. The first required paramter is shape followed by the value of number to fill the dimension.
tf.fill([1,2,3],5)
Unlike a variable , a variable’s value can be changed .However the variables data type and shape are fixed. We can create a variable as shown below.
tf.Variable([1,2,3,4,5],dtype=tf.float32)