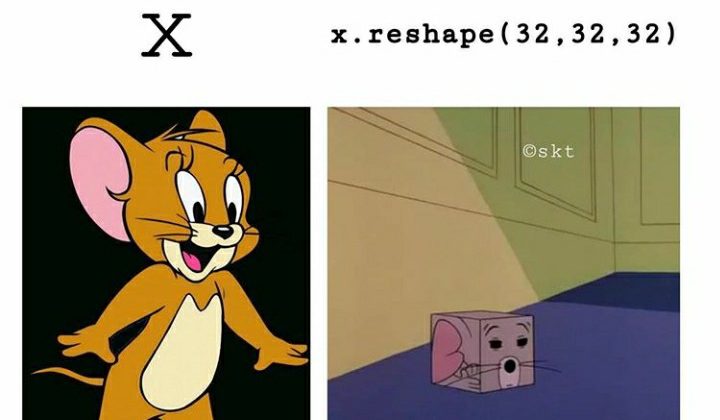

NumPy is unarguably one of the most essential libraries in Python. It provides support for high performance multi-dimensional array computation. And before this article gets any more boring, read the next line.
WARNING: This article is full of NumPy memes. Move ahead only if you want to see the best compilation of NumPy memes.
Importing NumPy
import numpy as np
NumPy arrays are the most important component of the entire NumPy library.
You can think of the np array as a homogenous list. What that means is that np arrays store data with the same data type i.e. you can’t store both integer and string in the same array. They are of the type ndarray.
NumPy arrays, are much different than a traditional Python List.
NumPy are preferred over Python lists, as they are faster, more compact, and consume lesser space than the list. Not only that, they are much more convenient to use than lists.
Let’s take a quick look over some of the common NumPy functions and attributes of the ndarray.
np.arange()
It generates a ndarray with values within a specified range.
Slicing NumPy arrays
arr[start:end:step]
Printing odd values from an array:
Reversing a NumPy array
Shape and Dimensions
We use arr.shape() to find the shape of the ndarray, and arr.ndim() to find the number of dimensions. Mentioning the ndmin argument while creating an array specifies the minimum number of dimensions the ndarray should have.
Reshaping the ndarray
We can reshape a ndarray to any desired shape without changing it’s data by using the .reshape() attribute.
Matrix Multiplication
We use the np.dot() function to multiply two ndarrays. Make sure to check the shape of your matrices before multiplying.
We can also make use of the matmul() function or simply a@b for multiplying two arrays.
Random module
Random module of the numpy library is a great way to generate random numbers and comes very handy.
random.rand() generates a random float between 0 and 1.
The below code generate a 2-D array with 3 rows, each row containing 3 random integers from 0 to 100:
ravel()
It returns a contiguous flattened array. Converts any multi-dimensional array into 1-dimension.
These were examples of some commonly used functions in NumPy.
The best resource to learn and understand the NumPy library is their documentation itself!
Here’s the link: https://numpy.org/doc/stable