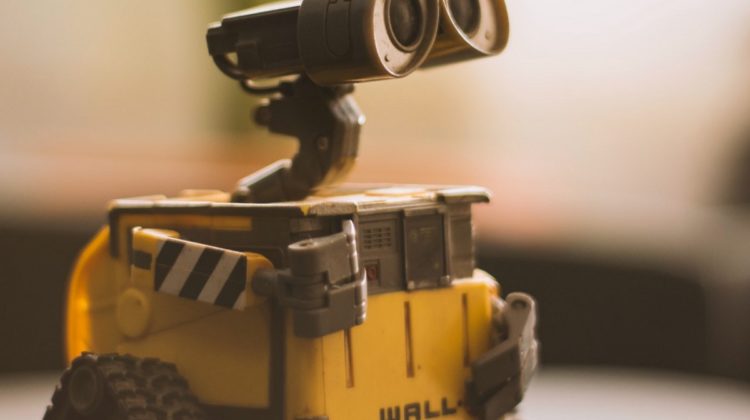
pip install opencv-python
- Importing OpenCV to your .py project:
import cv2
- Importing an Image and Viewing it:
You can close the window showing the image by pressing any button.
img = cv2.imread("<../Path/to/Image.Extension>")
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Note: OpenCV reads are in BGR color scheme instead of the usual RGB scheme.
rgb_img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
Note: From now, the image is referred to as
rgb_img
.
- Create a function to display images quickly
def viewImage(img, name_of_window):
cv2.namedWindow(name_of_window, cv2.WINDOW_NORMAL)
cv2.imshow(name_of_window, img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Cropping an Image:
To crop an image, we can directly provide width and height similar to slicing in Python.
# Syntax for cropping <image_name>[y:y+h, x:x+w]
crop = rgb_img[10:500, 500:2000]
viewImage(crop, "Cropped Image")
Resizing an Image:
For image scaling, we can use the cv2.resize()
function.
# Provide the % times of the original image you want to scale
scaling = 20#Change Width and Height
width = int(rgb_img.shape[1] * scaling / 100)
height = int(rgb_img.shape[0] * scaling / 100)
dim = (width,height)resized = cv2.resize(img,dim,interpolation = cv2.INTER_AREA)viewImage(resized, "Resized Image by 20%")
image.shape
outputs the height, width, and channels of an image.
Rotating an Image:
Images can be rotated clockwise and counterclockwise by applying a +ve
or-ve
angle rotation to it respectively.
(h, w, d) = rgb_img.shape
center_img = (w//2, h//2)
rotationMatrix = cv2.getRotationMatrix2D(center, 180, 1.0)
rotated = cv2.warpAffine(rgb_img, rotationMatrix, (w, h))viewImage(rotated, "Rotated Image 180 degrees")
Grayscaling and Thresholding an Image:
Grayscale is a black and white, single-channeled version of an Image. Whereas, Thresholding will turn all dark shades (less than 127 value) to 0 and all the bright shades to 255.
grey_img = cv2.cvtColor(rgb_img, cv2.COLOR_RGB2GRAY)
ret, threshold_img = cv2.threshold(gray_img,127,255,0)
viewImage(gray_img, "Grayscale Image")
viewImage(threshold_img, "Threshold Image")
Note: Parameter
ret
is unused.ret
stand for retVal which is used in Otsu’s Binarization. You can read about it here.
Blurring/Smoothing an Image:
There are multiple functions in OpenCV that can be used to control the Blurring and Smoothing of an Image. For the purpose of this blog, we will be using the Gaussian Blur
function.
The Gaussian Blur
function takes 3 parameters:
- Image you want to Blur.
- A tuple of 2 positive odd numbers. When increased, the blur effect increases.
- The sigmaX and sigmaY for the Gaussian Kernel. When set as 0, they’re automatically calculated from kernel size.
Gaussian Blur
is highly effective in removing noise from an image.
blurred = cv2.GaussianBlur(rgb_img, (61,61), 0)
viewImage(blurred, "Blurred Image")
Drawing a Bounding Box on an Image:
A bounding box is nothing but a rectangle or a square around an object. This creation is extensively used by many face detecting or object detecting applications.
output = rgb_img.copy()
cv2.rectangle(output, (2600,800), (4100, 2400), (0, 255, 255), 10)
viewImage(output, "Rectangle on an Image")
Parameters of cv2.rectangle()
are:
- Image import.
- x1,y1 — Top Left Corner.
- x2,y2 — Bottom Right Corner.
- Rectangle Color — Depends on the Image imported. (In our case RGB).
- Thickness of the production.
Similarly, we can draw lines using cv2.line
and add text using cv2.putText
on an Image.
Face Detection:
Face detection can be done by using inbuilt face_cascade
and detectMultiScale
function in OpenCV. We also make use of the CascadeClassifier
function.
image_path = "<../Path/To/Image.Extension>"
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
Haar feature-based cascade classifier can be read in the paper “Rapid Object Detection using a Boosted Cascade of Simple Features” by Paul Viola and Michael Jones.
image = cv2.imread(image_path)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, scaleFactor = 1.1,
minNeighbors = 5, minSize = (10,10)
)faces_detected = format(len(faces)) + "Face detected!"
print(face_detected)for(x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255,255,0), 2)viewImage(image, face_detected)
The detectMultiScale
function uses four parameters:
- Grayscale Image Input.
scaleFactor
compensates for the fact that some faces may be close or far away from the camera.- The detection algorithm uses a moving window to detect objects.
minNeighbors
defines how many objects are detected near the current one before it declares the face found. minSize
gives the size of each window.
Saving an Image
Finally, to save an Image, we can use the imwrite()
function.
image = cv2.imread("<../Path/To/Image.Extension>")
cv2.imwrite("../Export/to/Path.Extension", image)
Other functions can be read here.