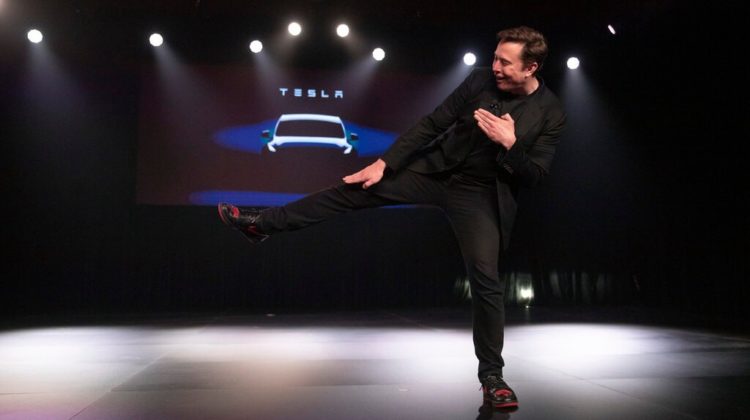
Predicting The Price of Tesla’s Stocks Using Machine Learning
Hi! In this article, I will show you how to write a python program that predicts the price of Tesla stocks using a machine learning technique called Long Short-Term Memory (LSTM).
The S&P 500, or Standard & Poor’s 500 index, is a market capitalization-weighted index of the 500 largest US palaces open. The index is considered to be the best indicator of wide-ranging US stocks.
US automotive company Tesla has officially been included in the S&P 500 Index as of December 21. With more than 730 percent appreciation in Tesla shares this year, the market value has increased to $ 658 billion. It has gained more than 70 percent since mid-November alone.
This value of Tesla is thought to be inflated. In this article, I will show you how to write a python program that predicts the price of Tesla’s stocks using a machine learning technique called Long Short-Term Memory (LSTM).
LSTMs are widely used for sequence prediction problems and have proven to be extremely effective. The reason they work so well is that LSTM is able to store past information that is important and forget the information that is not.
I will start by stating what I want this program to do. I want this program to predict the prices of Tesla Inc. stock 180 days in the future based off of the current Close price.
First I will write a description of the program. Then, I will import the libraries that will be used throughout this program.
I will get the stock quote for the company ‘Tesla Inc.’ using the companies stock ticker (TSLA) from July 1st, 2011 to December 28th, 2020.
I pulled the values from yahoo, but you can use “investing.com”.
Graph showing the closing stock price history of Tesla Inc. Then, create a new data frame with only the closing price and convert it to an array.
Then create a variable to store the length of the training data set. I want the training data set to contain about 85% of the data.
Now scale the data set to be valued between 0 and 1 inclusive, I do this because it is generally a good practice to scale your data before giving it to the neural network.
Create a training dataset containing the last 180-day closing price values we want to use to estimate the 181st closing price value.
So, the first column in dataset ‘x_train’ will contain values from a dataset from index 0 to index 179, and the second column will contain values from a dataset from index 1 to index 180.
The data set ‘Y_train’ is the 181st value found in index 180 for the first column and so on.
Now convert the independent train dataset ‘x_train’ and the dependent train dataset to ‘y_train’ NumPy sequences so that they can be used to train the LSTM model.
Create the scaled training data set.
Each LSTMs memory cell requires a 3D input. When an LSTM processes one input sequence of time steps, each memory cell will output a single value for the whole sequence as a 2D array.
Reshape the data to be 3-dimensional in the form [number of samples, number of time steps, and number of features]. The LSTM model is expecting a 3-dimensional data set.
Below is an example of defining a two hidden layer Stacked LSTM:
Build the LSTM model to have two LSTM layers with 50 neurons and two Dense layers, one with 25 neurons and the other with 1 neuron.
Compile the model using the mean squared error (MSE) loss function and the adam optimizer.
Create a test data set. Then convert the independent test data set ‘x_test’ to a NumPy array so it can be used for testing the LSTM model.
Now get the predicted values from the model using the test data.
Get the root mean squared error (RMSE), which is a good measure of how accurate the model is. A value of 0 would indicate that the models predicted values match the actual values from the test data set perfectly.
The lower the value the better the model performed. But usually, it is best to use other metrics as well to truly get an idea of how well the model performed.
Let’s plot and visualize the data.
Show the valid and predicted prices.
I want to test the model some more and get the predicted closing price value of Tesla Inc. for December 28, 2020 (12/28/2020).
So I will get the quote, convert the data to an array that contains only the closing price. Then I will get the last 180-day closing price and scale the data to be values between 0 and 1 inclusive.
After that, I will create an empty list and append the past 180-day price to it, and then convert it to a NumPy array and reshape it so that I can input the data into the model.
Last but not least, I will input the data into the model and get the predicted price.
Now let’s see what the actual price for that day was.
And the actual prices for 28/06/2020 to 12/29/2020.
What do you think of this rapid rise? Is Tesla’s stock really inflated? Or is this rise due to the pandemic effect?
Thanks for reading this article.
Referance
https://machinelearningmastery.com/stacked-long-short-term-memory-networks/