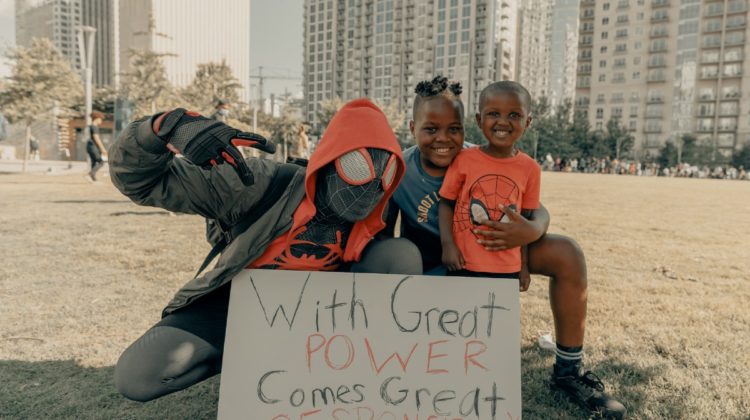
Lambda functions are very powerful items in the Python world that bridge the gap of whether or not you need to formally define a function or not. I would label the lambda function in Python as a sort of trump card for helping you to implement something quick and easy, without the formality of defining a function and any additional work that that would encompass. Remembering that “With great power comes great responsibility”, knowing when you can and when you shouldn’t use a lambda function is equally important.
If you are well onto your way of navigating the syntax and understanding how some of the core methods and functions work in Python, learning what and how the lambda function works may help to ease some of those unnecessarily tough problems in dealing with “simple functions”. It is always good practice to build all your code enclosed in functions, but sometimes you want to make something really simple and don’t want to have to go through the formality of defining a new function… This is where the lambda function comes in. Lambda functions allow you to declare an anonymous function with arguments and an expression. For simple routine manipulations, a lambda function can be an ideal option in contrast to building a standard function.
The devil in the detail is that overuse or improper use of lambda functions can lead to headaches down the road when it comes to troubleshooting as you may need to hunt up and down code to find an error and/or make changes/updates to code. Lambda functions should be used judiciously and where appropriate.
Basic Lambda Function Form and Syntax
- Variable to assign to — This is optional in how you want to implement the lambda function, but you can assign it to a variable and use that variable for further calculations and manipulations
- Function call — simply use lambda to implement the function
- Arguments for the function — You can have multiple arguments, but usually no more than two is a good rule of thumb for lambda functions. If you need more arguments, you might want to reconsider constructing a standard function instead
- Expression — Lambda functions are intended to be written in one line of code (hence why they can help simplify short, small bits of code)
- IFFE argument assignment — IFFE (immediately invoked function expression) arguments are used as the acronym implies to run the function with the arguments being these values. This section is optional as you can call the lambda function and assign the argument values more like a traditional function, as demonstrated in the next code block below.
You can see in the image above that executing the single line of the lambda function will yield 5 as does below with a different method of implementation.
x = (lambda x, y: x + y)
z = x(2,3)
print(z)
...
5
Different ways to implement lambda functions:
Due to the simplistic nature that lambda functions allow you to build a one-liner function and implement it, there are various different ways you can use them to make your code more functional. One way would be to use a lambda to set the key for sorting like below.
rank_list = sorted(score_mean.items(),
reverse=True,
key=lambda x: x[1])
So in the new list, named ‘rank_list’, we use the lambda function to sort based on the second element of the values for the dictionary, score_mean.
Another possible way to use it is as a simple function to apply in an iteration like below.
distance = (lambda x, y:
(1 - np.dot(x, y) / (np.linalg.norm(x) * np.linalg.norm(y))))
...for idx, vector_1 in enumerate(projected_vector):
article_distance[articles[idx]] = distance(vector_1, vector_2)
In the code block above, we are using the lambda to capture a cosine similarity calculation and then applying it later to find the distance between two vectors. We are doing this to each item in the loop and capturing the distances for manipulation later.
Limitations on Lambdas
As mentioned before using lambdas should be used judiciously and with a thought on clarity and necessity to ensure good code review and to facilitate updates in the future. Although lambdas act like normal functions there are a few limitations on them that you should consider before directly jumping straight into lambda functions:
- Since lambda functions are meant to be quick one-liners normal python statements can’t be used in the expression.
- Due to the functions technically being anonymous in nature they are not necessarily bound to a name.
- Limited complexity due to the items mentioned before: one statement, a single line, and probably not (or shouldn’t) include too many arguments
Wrap Up
Lambdas are a very useful tool to have in your bag. They allow for quick and easy functions to be implemented in your code but do have some limitations in what they can do. Think about when and why you are going to use them and why a normal function might not be optimal. As I mentioned at the start “With great power, Come great responsibility!” The same applies to Lambdas. If you have any questions, comments, or feedback let me know! Thanks.