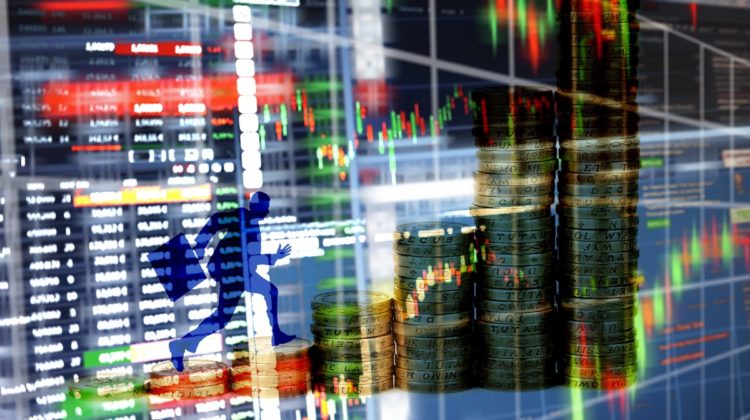
The above plot shows an example on the EURUSD hourly data plotted with the Equilibrium Indicator in blue. The full code can be find below:
def ema(Data, alpha, lookback, what, where):# alpha is the smoothing factor
# window is the lookback period
# what is the column that needs to have its average calculated
# where is where to put the exponential moving averagealpha = alpha / (lookback + 1.0)
beta = 1 - alpha# First value is a simple SMA
Data = ma(Data, lookback, what, where)# Calculating first EMA
# Calculating the rest of EMA
Data[lookback + 1, where] = (Data[lookback + 1, what] * alpha) + (Data[lookback, where] * beta)
for i in range(lookback + 2, len(Data)):
try:
Data[i, where] = (Data[i, what] * alpha) + (Data[i - 1, where] * beta)except IndexError:
def deleter(Data, index, times):
pass
return Datafor i in range(1, times + 1):
Data = np.delete(Data, index, axis = 1)
return Datadef jump(Data, jump):
Data = Data[jump:, ]
return Data
def equilibrium(Data, lookback, close, where):# Calculating the moving average
Data = ma(Data, lookback, close, where)
Data = jump(Data, lookback)# Calculating the distance from the moving average
Data[:, where + 1] = Data[:, close] - Data[:, where]# Calculating the Exponential Moving Average of the Equilibrium
Data = ema(Data, 2, lookback, where + 1, where + 2)
Data = jump(Data, lookback)
Data = deleter(Data, where, 2)return Data
# Using the function
my_data = equilibrium(Data, 5, 3, 4)